As a blooming Python developer who has just written some Python code, you’re immediately faced with the important question, “how do I run it?” Before answering that question, let’s back up a little to cover one of the fundamental elements of Python.
An Interpreted Language
Python is an interpreted programming language, meaning Python code must be run using the Python interpreter.
Traditional programming languages like C/C++ are compiled, meaning that before it can be run, the human-readable code is passed into a compiler (special program) to generate machine code – a series of bytes providing specific instructions to specific types of processors. However, Python is different. Since it’s an interpreted programming language, each line of human-readable code is passed to an interpreter that converts it to machine code at run time.
So to run Python code, all you have to do is point the interpreter at your code.
Different Versions of the Python Interpreter
It’s critical to point out that there are different versions of the Python interpreter. The major Python version you’ll likely see is Python 2 or Python 3, but there are sub-versions (i.e. Python 2.7, Python 3.5, Python 3.7, etc.). Sometimes these differences are subtle. Sometimes they’re dramatically different. It’s important to always know which Python version is compatible with your Python code.
Run a script using the Python interpreter
To run a script, we have to point the Python interpreter at our Python code…but how do we do that? There are a few different ways, and there are some differences between how Windows and Linux/Mac operating systems do things. For these examples, we’re assuming that both Python 2.7 and Python 3.5 are installed.
Our Test Script
For our examples, we’re going to start by using this simple script called test.py.
test.py print(“Aw yeah!”)'
How to Run a Python Script on Windows
The py Command
The default Python interpreter is referenced on Windows using the command py. Using the Command Prompt, you can use the -V option to print out the version.
Command Prompt
> py -V
Python 3.5
You can also specify the version of Python you’d like to run. For Windows, you can just provide an option like -2.7 to run version 2.7.
Command Prompt> py -2.7 -V
Python 2.7
On Windows, the .py extension is registered to run a script file with that extension using the Python interpreter. However, the version of the default Python interpreter isn’t always consistent, so it’s best to always run your scripts as explicitly as possible.
To run a script, use the py command to specify the Python interpreter followed by the name of the script you want to run with the interpreter. To avoid using the full file path to your script (i.e. X:\General Assembly\test.py), make sure your Command Prompt is in the same directory as your Python script file. For example, to run our script test.py, run the following command:
Command Prompt > py -3.5 test.py
Aw yeah!
Using a Batch File
If you don’t want to have to remember which version to use every time you run your Python program, you can also create a batch file to specify the command. For instance, create a batch file called test.bat with the contents:
test.bat@echo off
py -3.5 test.py
This file simply runs your py command with the desired options. It includes an optional line “@echo off” that prevents the py command from being echoed to the screen when it’s run. If you find the echo helpful, just remove that line.
Now, if you want to run your Python program test.py, all you have to do is run this batch file.
Command Prompt > test.bat
Aw yeah!
How to Run a Python Script on Linux/Mac
The py Command
Linux/Mac references the Python interpreter using the command python. Similar to the Windows py command, you can print out the version using the -V option.
Terminal$ python -V
Python 2.7
For Linux/Mac, specifying the version of Python is a bit more complicated than Windows because the python commands are typically a bunch of symbolic links (symlinks) or shortcuts to other commands. Typically, python is a symlink to the command python2, python2 is a symlink to a command like python2.7, and python3 is a symlink to a command like python3.5. One way to view the different python commands available to you is using the following command:
Terminal$ ls -1 $(which python)* | egrep ‘python($|[0-9])’ | egrep -v config
/usr/bin/python
/usr/bin/python2
/usr/bin/python2.7
/usr/bin/python3
/usr/bin/python3.5
To run our script, you can use the Python interpreter command and point it to the script.
Terminal $ python3.5 test.py
Aw yeah!
However, there’s a better way of doing this.
Using a shebang
First, we’re going to modify the script so it has an additional line at the top starting with ‘#!’ and known as a shebang (shebangs, shebangs…).
test.py#!/usr/bin/env python3.5
print(“Aw yeah!”)
This special shebang line tells the computer how to interpret the contents of the file. If you executed the file test.py without that line, it would look for special instruction bytes and be confused when all it finds is a text file. With that line, the computer knows that it should run the contents of the file as Python code using the Python interpreter.
You could also replace that line with the full file path to the interpreter:
#!/usr/bin/python3.5
However, different versions of Linux might install the Python interpreter in different locations, so this method can cause problems. For maximum portability, I always use the line with /usr/bin/env that looks for the python3.5 command by searching the PATH environment variable, but the choice is up to you.
Next, we’re going to set the permissions of this file to be Python executable with this command:
Terminal $ chmod +x test.py
Now we can run the program using the command ./test.py!
Terminal $ ./test.py
Aw yeah!
Pretty sweet, eh?
Run the Python Interpreter Interactively
One of the awesome things about Python is that you can run the interpreter in an interactive mode. Instead of using your py or python command pointing to a file, run it by itself, and you’ll get something that looks like this:
Command Prompt> py
Python 3.7.3 (v3.7.3:ef4ec6ed12, Mar 25 2019, 21:26:53) [MSC v.1916 32 bit (Intel)] on win32
Type "help", "copyright", "credits" or "license" for more information.
>>>
Now you get an interactive command prompt where you can type in individual lines of Python!
Command Prompt (Python Interpreter)>>> print(“Aw yeah!”)
Aw yeah!
What’s great about using the interpreter in interactive mode is that you can test out individual lines of Python code without writing an entire program. It also remembers what you’ve done, just like in a script, so things like functions and variables work the exact same way.
Command Prompt (Python Interpreter)>>> x = "Still got it."
>>> print(x)
Still got it.
How to Run a Python Script from a Text Editor
Depending on your workflow, you may prefer to run your Python program or Python script file directly from your text editor. Different text editors provide fancy ways of doing the same thing we’ve already done — pointing the Python interpreter at your Python code. To help you along, I’ve provided instructions on how to do this in four popular text editors.
- Notepad++
- VSCode
- Sublime Text
- Vim
1. Notepad++
Notepad++ is my favorite general purpose text editor to use on Windows. It’s also super easy to run a Python program from it.
Step 1: Press F5 to open up the Run… dialogue
Step 2: Enter the py command like you would on the command line, but instead of entering the name of your script, use the variable FULL_CURRENT_PATH like so:
py -3.5 -i "$(FULL_CURRENT_PATH)"
You’ll notice that I’ve also included a -i option to our py command to “inspect interactively after running the script”. All that means is it leaves the command prompt open after it’s finished, so instead of printing “Aw yeah!” and then immediately quitting, you get to see the Python program’s output.
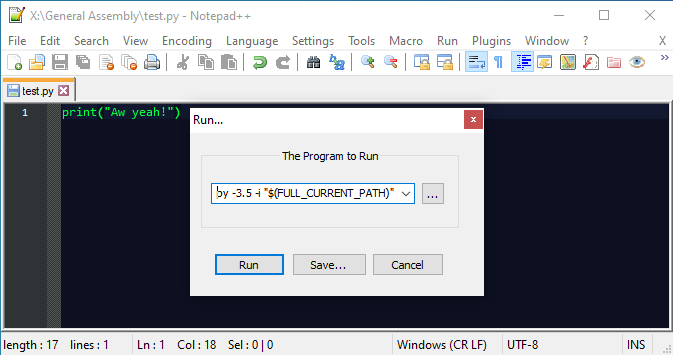
Step 3: Click Run

2. VSCode
VSCode is a Windows text editor designed specifically to work with code, and I’ve recently become a big fan of it. Running a Python program from VSCode is a bit complicated to set it up, but once you’ve done that, it works quite nicely.

Step 1: Go to the Extensions section by clicking this symbol or pressing CTRL+SHIFT+X.
Step 2: Search and install the extensions named Python and Code Runner, then restart VSCode.
Step 3: Right click in the text area and click the Run Code option or press CTRL+ALT+N to run the code.
Note: Depending on how you installed Python, you might run into an error here that says ‘python’ is not recognized as an internal or external command. By default, Python only installs the py command, but VSCode is quite intent on using the python command which is not currently in your PATH. Don’t worry, we can easily fix that.
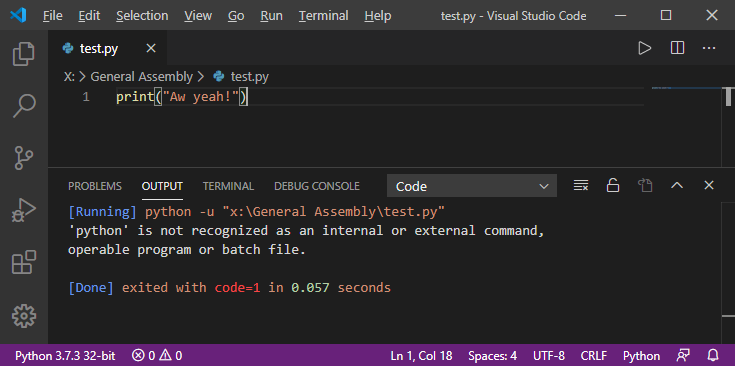
Step 3.1: Locate your Python installation binary or download another copy from www.python.org/downloads. Run it, then select Modify.
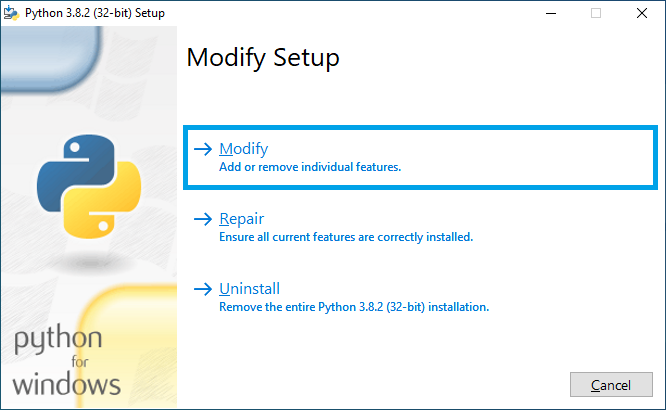
Step 3.2: Click next without modifying anything until you get to the Advanced Options, then check the box next to Add Python to environment variables. Then click Install, and let it do its thing.
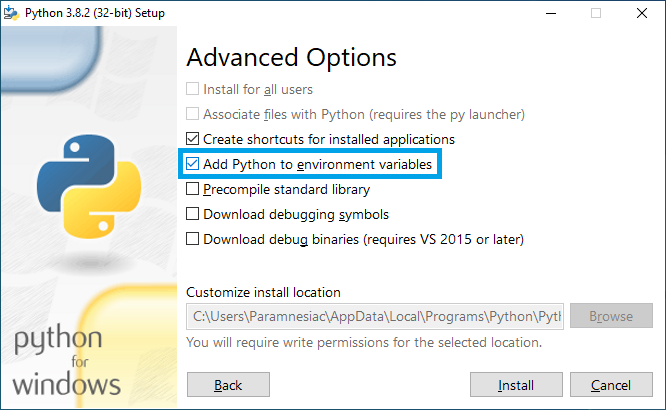
Step 3.3: Go back to VSCode and try again. Hopefully, it should now look a bit more like this:
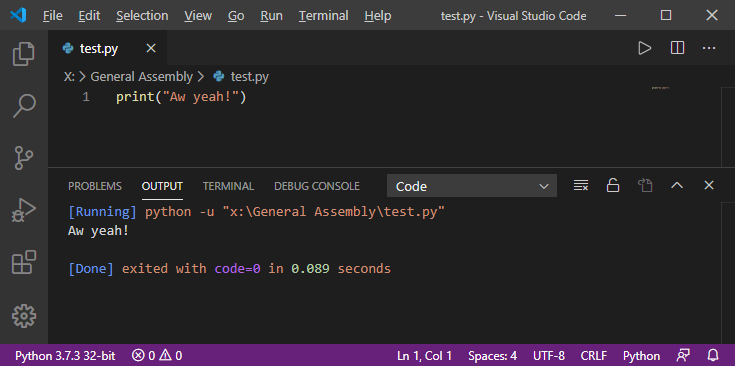
3. Sublime Text
Sublime Text is a popular text editor to use on Mac, and setting it up to run a Python program is super simple.
Step 1: In the menu, go to Tools → Build System and select Python.
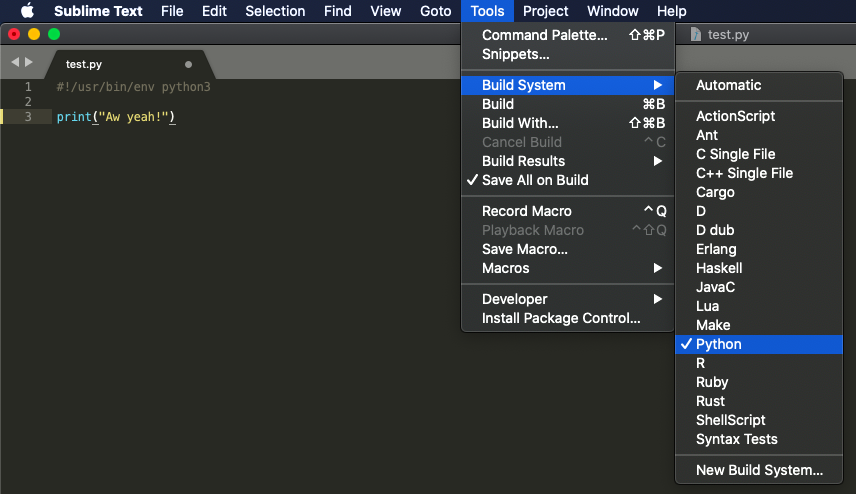
Step 2: Press command ⌘ +b or in the menu, go to Tools → Build.
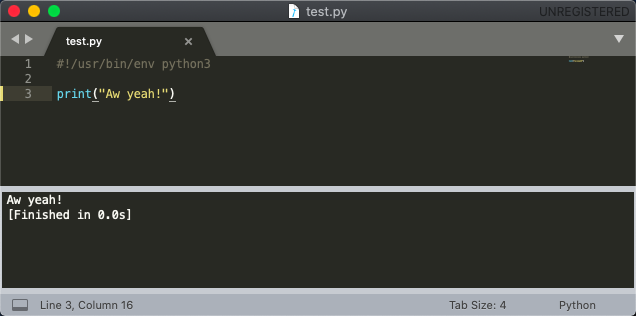
4. Vim
Vim is my text editor of choice when it comes to developing on Linux/Mac operating systems, and it can also be used to easily run a Python program.
Step 1: Enter the command :w !python3 and hit enter.
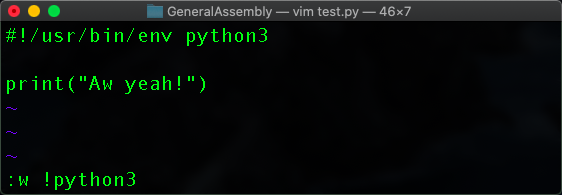
Step 2: Profit.
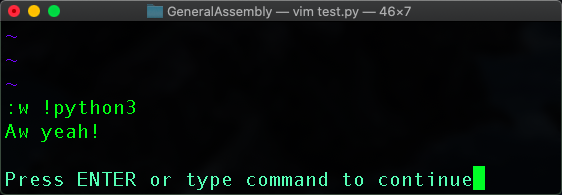
Now that you can successfully run your Python code, you’re well on your way to speaking parseltongue!