What is Python?: An Introduction
Python is one of the most popular and user-friendly programming languages out there. As a developer who’s learned a number of programming languages, Python is one of my favorites due to its simplicity and power. Whether I’m rapidly prototyping a new idea or developing a robust piece of software to run in production, Python is usually my language of choice.
The Python programming language is ideal for folks first learning to program. It abstracts away many of the more complicated elements of computer programming that can trip up beginners, and this simplicity gets you up-and-running much more quickly!
For instance, the classic “Hello world” program (it just prints out the words “Hello World!”) looks like this in C:
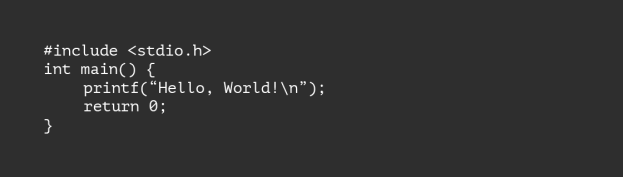
However, to understand everything that’s going on, you need to understand what #include means (am I excluding anyone?), how to declare a function, why there’s an “f” appended to the word “print,” etc., etc.
Not only is this an easier starting point, but as the complexity of your Python programming grows, this simplicity will make sure you’re spending more time writing awesome code and less time tracking down bugs!
Since Python is popular and open-source, there’s a thriving community of Python application developers online with extensive forums and documentation for whenever you need help. No matter what your issue is, the answer is usually only a quick Google search away.
If you’re new to programming or just looking to add another language to your arsenal, I would highly encourage you to join our community.
What Type of Language is Python?
Named after the classic British comedy troupe Monty Python, Python is a general-purpose, interpreted, object-oriented, high-level programming language with dynamic semantics. That’s a bit of a mouthful, so let’s break it down.
General-Purpose
Python is a general-purpose language which means it can be used for a wide variety of development tasks. Unlike a domain-specific language that can only be used for specific types of applications (think JavaScript and HTML/CSS for web development), a general-purpose language like Python can be used for:
Web applications: Popular frameworks like the Django web application and Flask are written in Python.
Desktop applications: The Dropbox client is written in Python.
Scientific and numeric computing: Python is the top choice for data science and machine learning.
Cybersecurity: Python is excellent for data analysis, writing system scripts that interact with an operating system, and communicating over network sockets.
Interpreted
Python is an interpreted language, meaning Python program code must be run using the Python interpreter.
Traditional programming languages like C/C++ are compiled, meaning that before it can be run, the human-readable code is passed into a compiler (special program) to generate machine code — a series of bytes providing specific instructions to specific types of processors. However, Python is different. Since it’s an interpreted programming language, each line of human-readable code is passed to an interpreter that converts it to machine code at run time.
In other words, instead of having to go through the sometimes complicated and lengthy process of compiling your code before running it, you just point the Python interpreter at your code, and you’re off!
Part of what makes an interpreted language great is how portable it is. Compiled languages must be compiled for the specific type of computer they’re run on (i.e. think your phone vs. your laptop). For Python, as long as you’ve installed the interpreter for your computer, the exact same code will run almost anywhere!
Object-Oriented
Python is an Object-Oriented Programming (OOP) language which means that all of its elements are broken down into things called objects. A Python object is very useful for software architecture and often makes it simpler to write large, complicated applications.
High-Level
Python is a high-level language which really just means that it’s simpler and more intuitive for a human to use. Low-level languages such as C/C++ require a much more detailed understanding of how a computer works. With a high-level language, many of these details are abstracted away to make your life easier.
For instance, say you have a list of three numbers — 1, 2, and 3 — and you want to append the number 4 to that list. In C, you have to worry about how the computer uses memory, understands different types of variables (i.e., an integer vs. a string), and keeps track of what you’re doing.
Implementing this in C code is rather complicated:
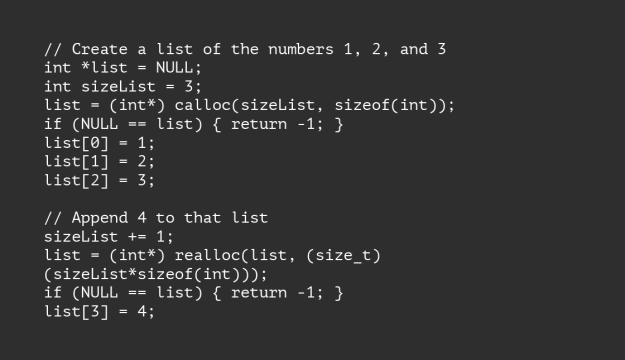
However, implementing this in Python code is much simpler:
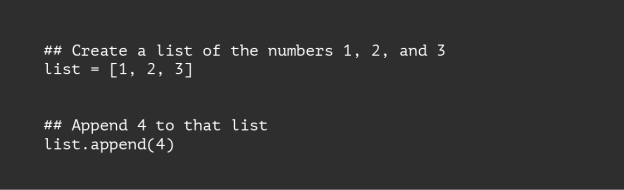
Since a list in Python is an object, you don’t need to specifically define what the data structure looks like or explain to the computer what it means to append the number 4. You just say “list.append(4)”, and you’re good.
Under the hood, the computer is still doing all of those complicated things, but as a developer, you don’t have to worry about them! Not only does that make your code easier to read, understand, and debug, but it means you can develop more complicated programs much faster.
Dynamic Semantics
Python uses dynamic semantics, meaning that its variables are dynamic objects. Essentially, it’s just another aspect of Python being a high-level language.
In the list example above, a low-level language like C requires you to statically define the type of a variable. So if you defined an integer x, set x = 3, and then set x = “pants”, the computer will get very confused. However, if you use Python to set x = 3, Python knows x is an integer. If you then set x = “pants”, Python knows that x is now a string.
In other words, Python lets you assign variables in a way that makes more sense to you than it does to the computer. It’s just another way that Python programming is intuitive.
It also gives you the ability to do something like creating a list where different elements have different types like the list [1, 2, “three”, “four”]. Defining that in a language like C would be a nightmare, but in Python, that’s all there is to it.
It’s Popular. Like, Super Popular.
Being so powerful, flexible, and user-friendly, the Python language has become incredibly popular. Python’s popularity is important for a few reasons.
Python Programming is in Demand
If you’re looking for a new skill to help you land your next job, learning Python is a great move. Because of its versatility, Python is used by many top tech companies. Netflix, Uber, Pinterest, Instagram, and Spotify all build their applications using Python. It’s also a favorite programming language of folks in data science and machine learning, so if you’re interested in going into those fields, learning Python is a good first step. With all of the folks using Python, it’s a programming language that will still be just as relevant years from now.
Dedicated Community
Python developers have tons of support online. It’s open-source with extensive documentation, and there are tons of articles and forum posts dedicated to it. As a professional Python developer, I rely on this community everyday to get my code up and running as quickly and easily as possible.
There are also numerous Python libraries readily available online! If you ever need more functionality, someone on the internet has likely already written a library to do just that. All you have to do is download it, write the line “import <library>”, and off you go. Part of Python’s popularity in data science and machine learning is the widespread use of its libraries such as NumPy, Pandas, SciPy, and TensorFlow.
Conclusion
Python is a great way to start programming and a great tool for experienced developers. It’s powerful, user-friendly, and enables you to spend more time writing badass code and less time debugging it. With all of the libraries available, it will do almost anything you want it to.
The final answer to the question “What is Python”? Awesome. Python is awesome.