Our Software Engineering Career Guide Series:
- Software Engineering Overview
- Software Engineering Skills & Tools <– You Are Here
- Software Engineering Education & Training
- Software Engineering Career Path & Salary
- Software Engineering Portfolio & Resume
- Software Engineering Interviewing
So, you’re interested in becoming a software engineer? Great choice. As a software engineer, you’ll be at the forefront of innovation, shaping the digital landscape of tomorrow. Chief software engineer duties include designing, developing, and maintaining software systems that power virtually everything — from mobile apps to complex enterprise solutions.
So, naturally, the demand for skilled software engineers is soaring, with industries like artificial intelligence, cybersecurity, and cloud computing leading the charge. While traditional “Big Tech” companies like Google, Amazon, and Facebook are constantly on the lookout for top talent, every industry — from healthcare and retail to entertainment and finance — relies on software solutions to innovate and stay competitive.
As you gain experience and expertise, you’ll find a multitude of avenues to apply your software engineering skills and make a meaningful impact in areas that align with your interests. And with salaries typically ranging from $100,000 to $150,000, investing in your education to shore up your skills and learn the tools of the trade promises a rapid return on investment.
But what does it take to thrive in this dynamic field? Transitioning into the role of a software engineer requires a unique blend of technical prowess, problem-solving abilities, and creativity. Let’s delve into the essential skills and tools you’ll need to master to succeed in this exciting career path.
Software Engineering Technical Skills
The technical skills required for software engineers include:
- Designing: You’ll put your eye for design to work creating user-friendly interfaces and architecting scalable, maintainable software systems.
- Programming: Competency in programming languages such as Python, Java, and C++ enables you to translate ideas into efficient and scalable code.
- Software development: Using software development methodologies like Agile or Scrum, you’ll collaborate and manage projects with your development teams to systematically evolve software within project requirements and goals.
Software testing: Equally vital is proficiency in software testing methodologies to ensure the reliability and functionality of your applications through rigorous testing processes, reducing the likelihood of bugs and enhancing your end user experience.
Debugging: Critical for identifying and rectifying errors in code, debugging skills help you identify and resolve software defects swiftly, maintaining the integrity of the code.
Database administration: Data integrity and accessibility hinges on efficient data management and storage, which will make your projects easier to scale.
By learning these software developer technical skills, you’ll be able to work on highly productive product development teams and deliver high-quality, robust software solutions.
Software Engineering Soft Skills
Being a great software engineer isn’t just about your technical chops — it’s also about how you relate to others. In today’s rapidly evolving business landscape, where technical needs are in constant flux, the importance of soft skills in software engineering cannot be overstated.
According to recent surveys, 92% of companies believe durable soft skills matter even more than technical skills in today’s business world.
Cultivating empathy, communication, and adaptability — much in the way you’d learn to play a musical instrument or learn the finesse of a sport — empowers you to adapt to emerging technologies and tackle diverse challenges with confidence and agility.
Before you interview, consider how you might demonstrate software developer soft skills like:
- Communication: You’ll articulate complex technical concepts to non-technical stakeholders, ensuring alignment and understanding across teams.
- Organization: You’ll manage multiple tasks and priorities, keeping projects on track and meeting deadlines consistently.
- Problem-solving: You’ll approach challenges creatively and methodically, driving innovation and facilitating smooth resolutions.
- Attention to detail: You’ll meticulously review and test code, preventing costly errors and maintaining the integrity of your code.
As the day begins and you gather with your team for the morning stand-up meeting, active listening sets the tone for a positive work environment. Your ability to empathize and communicate constructively fosters collaboration and camaraderie.
Whether brainstorming new features, reviewing code, or meeting with clients, your adept communication skills ensure effective collaboration and successful project outcomes. While much of your work may be independent, it’s these interpersonal interactions that underpin your company’s success.
Demonstrating proficiency in these software developer basic skills, in addition to your technical abilities, will make you a standout candidate in any job interview.
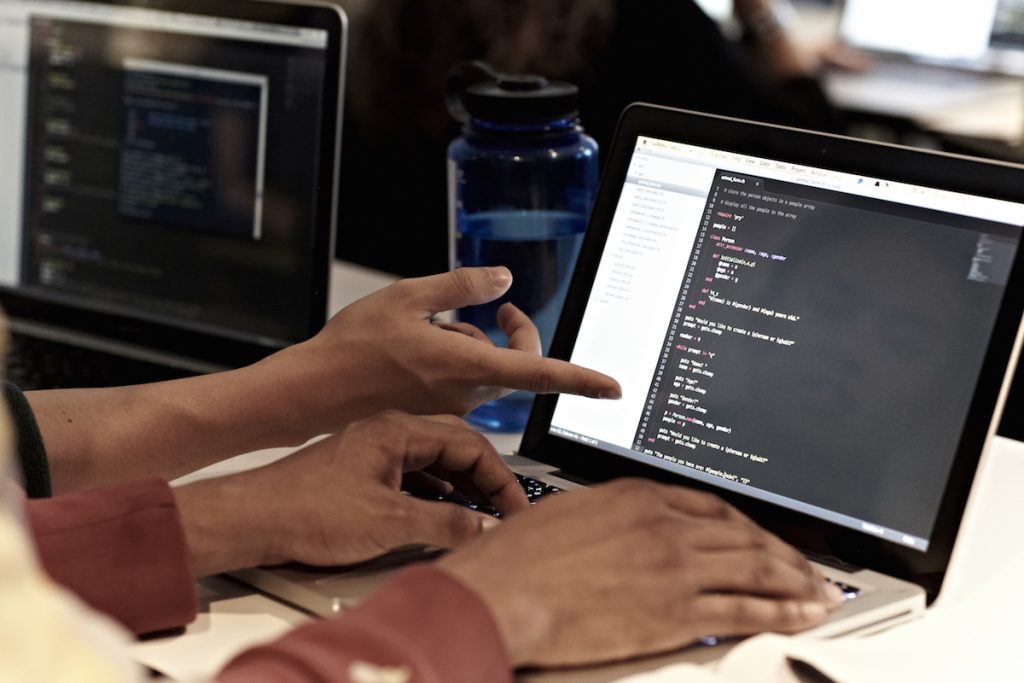
How To Advance Your Software Engineering Skills Toward Mastery
Enhancing your software engineering skills requires a multifaceted approach. While formal education gives you a solid theoretical foundation, mastery comes with gaining hands-on experience with programming languages, frameworks, and software development tools.
- Practical experience: Internships, freelance work, or personal projects engage you in real-world projects, allowing you to apply theoretical concepts to concrete problems, hone your problem-solving abilities, and deepen your understanding of programming languages and frameworks.
- Programming languages and frameworks: Online tutorials, coding challenges, and project-based learning enable dedicated time to enhance your developer skills through in-depth exploration of programming languages (like Python, Java, and JavaScript) and frameworks (like React, Angular, and Django).
- Tools and technologies: Explore tutorials and documentation on version control systems like Git, collaboration platforms like GitHub, and integrated development environments (IDEs) such as Visual Studio Code. By familiarizing yourself with software engineer tools and technologies, you’ll understand modern software engineering workflows and find ways to streamline your development process.
- Learning resources: Fortunately, a wealth of resources is available to support your journey in improving software engineering skills. In addition to offering part-time and full-time immersive bootcamps, General Assembly also has free courses, workshops, and learning resources covering a wide range of topics, from beginner to advanced levels. Additionally, developer communities and forums like StackOverflow provide valuable insights and support from experienced professionals that accelerate your learning.
- Continuous improvement: Most importantly, embracing a mindset of continuous improvement will position you for success in an ever-evolving field of software engineering. You can stay updated on industry trends and emerging technologies by attending industry conferences, participating in coding challenges, and seeking feedback from peers and mentors to identify areas for growth.
- More Resources for Learning Software Development: Additional resources beyond formal education can accelerate learning and deepen understanding over time by offering diverse perspectives, practical insights, and invaluable feedback.
- YouTube channels: Explore YouTube channels dedicated to software development, where industry experts share tutorials, tips, and best practices. Channels like Traversy Media, The Net Ninja, and Programming with Mosh offer comprehensive guides on programming languages, frameworks, and tools, catering to learners of all levels.
- Forums: Participate in online forums and communities like Stack Overflow, Reddit’s r/programming, and Hashnode, where developers congregate to discuss coding challenges, seek advice, and share knowledge. Engaging with these forums provides opportunities to learn from experienced professionals, troubleshoot issues, and expand your network within the software development community.
- Blogs and articles: Follow blogs and read articles authored by software engineers and industry thought leaders to stay updated on the latest trends, technologies, and best practices in software development. Platforms like Medium, DEV Community, and Towards Data Science publish insightful articles covering a range of topics, from software architecture best practices to design trends.
- Open-source projects on GitHub: Contribute to open-source projects on platforms like GitHub to gain practical experience, collaborate with other developers, and showcase your skills to potential employers. By actively participating in open-source communities, you’ll refine your coding abilities while honing soft skills like teamwork, communication, and project management.
- Mentorship: Experienced industry mentors provide personalized feedback, guidance, and support tailored to your individual learning goals. By proactively seeking out field experts, you’ll gain shortcuts to overcome obstacles and direction on which areas of study and experience are worthy of your focus.
Software Engineering Tools: A Partial List
In the dynamic landscape of software development, you’ll rely on various tools to enhance your productivity, collaboration, and innovation.
Here’s what some of the top software engineering tools of the trade will allow you to do:
Developer workflow tools
Track changes in your code, collaborate with team members, and manage different versions of your software projects.
Plan and track your software development projects, organize tasks, and communicate with your team.
Web development frameworks
Build dynamic, interactive user interfaces for your web applications, leveraging React’s component-based architecture and efficient rendering.
Develop robust and scalable web applications rapidly, leveraging Django’s clean design, built-in security features, and extensive libraries.
Programming languages
Write code for web development, data analysis, machine learning, and more, leveraging Python’s simplicity, versatility, and extensive ecosystem.
Develop enterprise-level applications and Android apps, using Java’s platform independence, object-oriented principles, and rich standard library.
Compliance databases
Store and manage unstructured data for modern applications, benefiting from MongoDB’s scalability, flexibility, and ease of use.
Handle relational databases for enterprise-grade applications, leveraging Oracle’s reliability, security features, and comprehensive support.
Containerization solutions
Automate the deployment, scaling, and management of your containerized applications, ensuring seamless integration and efficient resource use.
Package and distribute your applications in lightweight containers, enabling consistent deployment across different environments and simplifying the development process.
Testing methods
Verify the functionality, integration, and performance of software components and systems with unit, integration, and system methodology to ensure quality and reliability throughout your development lifecycle.
DevOps principles
Implement automated pipeline development and testing to streamline your development workflows with continuous integration, delivery, and quality assurance.
Microservices architecture
Design and develop modular and scalable CRM or payment processing applications composed of loosely coupled services, enabling agility and resilience in complex systems.
Cloud services
Leverage cloud services to deploy, scale, and manage your applications with flexibility, reliability, and cost-efficiency.
Artificial intelligence
Accelerate your coding process with AI-powered suggestions that assist in writing code faster and more efficiently based on natural language prompts.
Generate human-like text responses and code comments, leveraging AI-powered language models to streamline documentation and communication in your projects.
Boost your coding productivity with AI-driven code completions, predicting context-aware suggestions to improve your coding workflow and code quality.
By incorporating these tools into your software engineering projects, you’ll enhance your skills, streamline your workflows, and drive innovation.
Wondering if a career in software engineering is a good move? Sign up for our next info session to learn more.